Let’s build a very useful and flexible detector script, that you can easily use to detect if some body enters the specific space. Then using UnityEvnts system you will be able to flexibly notify other GameObject about this fact. The created detector allows you to use it in various situations in the future without writing any line of code.
Resources
The final project you can find on my GitLab. If you prefer video tutorial text, you can watch it on my YouTube channel.
Detector script
Let’s have a look on the following detector script. The script is a typical MonoBehaviour script and it requires Collider component be able to detect if any other object interacts with the collider. In that case that the script detects any interaction it broadcasts particular events trough UnityEvents system.
using UnityEngine; using UnityEngine.Events; namespace Commons { [RequireComponent(typeof(Collider))] public class Detector : MonoBehaviour { public UnityEvent<Collider> onTriggerEnter; public UnityEvent<Collider> onTriggerStay; public UnityEvent<Collider> onTriggerExit; private void OnTriggerEnter(Collider other) { onTriggerEnter?.Invoke(other); } private void OnTriggerStay(Collider other) { onTriggerStay?.Invoke(other); } private void OnTriggerExit(Collider other) { onTriggerExit?.Invoke(other); } } }
Receiver script
Now it’s time to implement a MonoBehavior with ability to handle the events emitted by Detector. The only requirement is that the receiver MonoBehaviour has to have implemented a public method with the same parameters as emitted events have.
using UnityEngine; namespace Commons { public class DummyReceiver : MonoBehaviour { public void ListenOnTriggerEnter(Collider other) { Debug.Log("ListenOnTriggerEnter"); } public void ListenOnTriggerStay(Collider other) { Debug.Log("ListenOnTriggerStay"); } public void ListenOnTriggerExit(Collider other) { Debug.Log("ListenOnTriggerEnter"); } } }
Putting it together – UnityEvent system
It’s time to put everything together. Create a sphere and add Rigidbody component to it. Then create two empty game objects – one for Detector and one for Receiver. Add some collider (for example BoxCollider) and the cerated Detector script to the Detector GameObject. Please consider to set IsTrigger=ture flag of the crated collider.
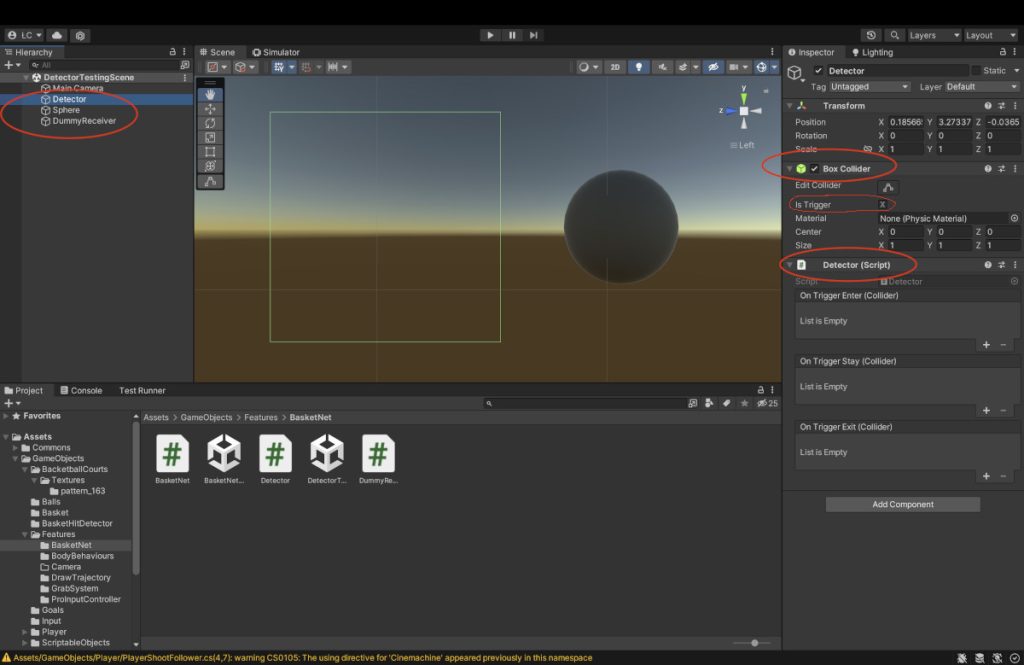
Then add DummyReceiver script to the Receiver GameObejct. It allows you to handle events generated by Detector.
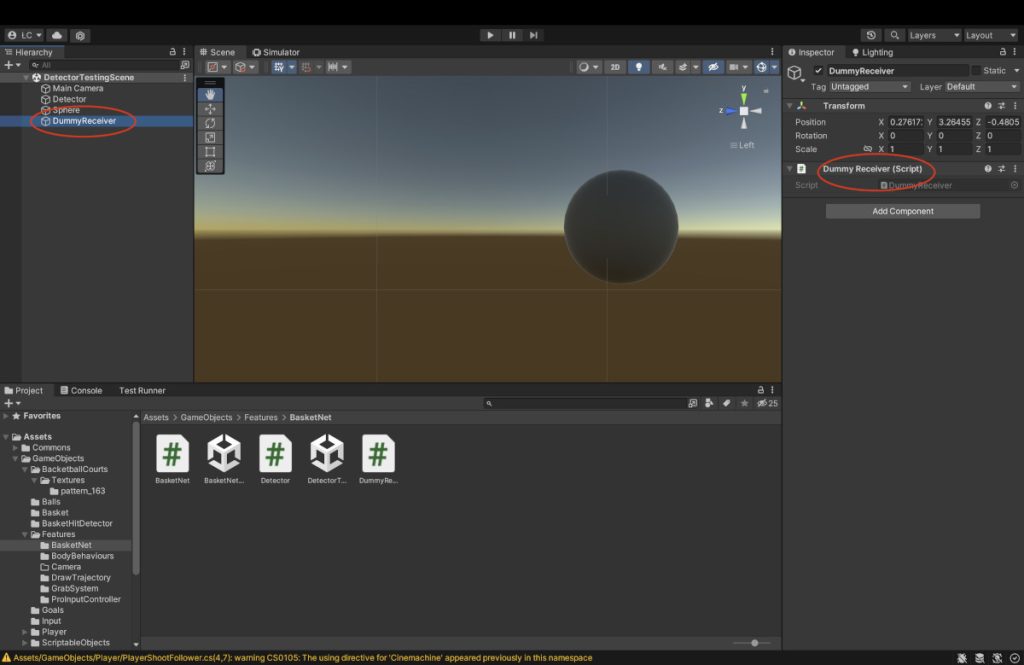
And now the most interesting part, which is event listeners configuration using UnityEvent system. It’s sounds complicated but it’s actually very simple. Just select Detector GameObject and add event listeners by clicking “+” icon. Drag DummyReceiver GameObejct into the property inspector field (1) and choose DummyReceiver’s event handlers as it is shown below (2,3,4).
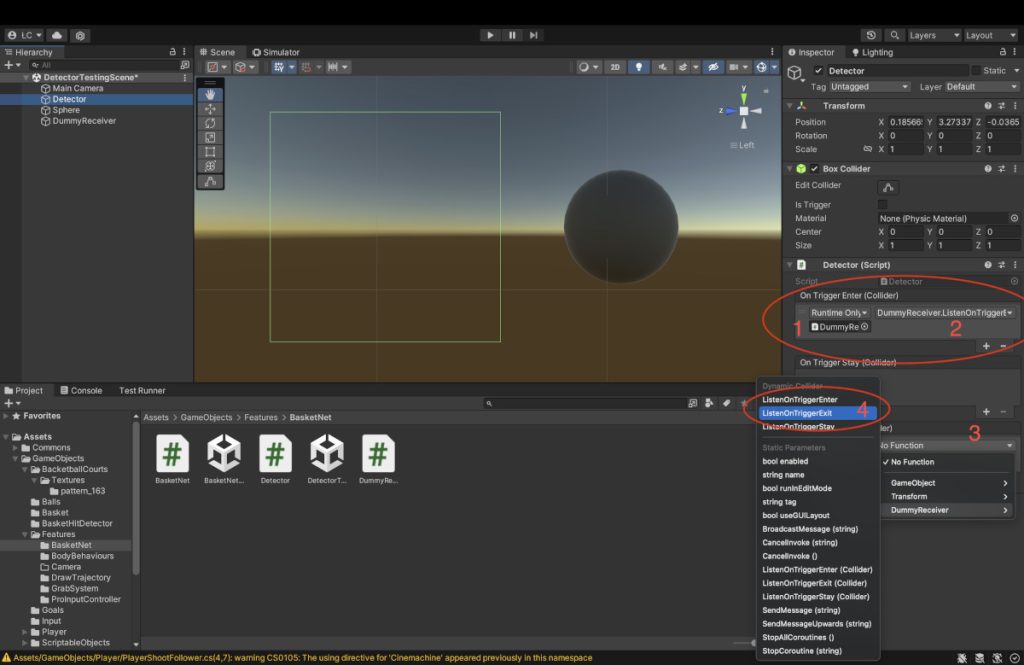
If you have done everything correctly the created detector should work. Run the simulation and test the behaviour of the detector by moving the Sphere in and out of the detector’s collider.
That’s all what I’ve prepared for you in this tutorial, if I helped you, please consider sharing this post to help me gain a wider audience.
Thanks and I hope to see you in my next tutorial.